Unlocking the Mysteries of User Not Authorized Error
Common Causes of User Not Authorized Error
1. Incorrect login credentials: Double-check that you are entering the correct username and password for your account.
2. Expired or revoked access: Ensure that your account still has the necessary permissions to access the resource you are trying to reach.
3. Browser cache issues: Clear your browser cache to eliminate any stored data that may be causing conflicts with your authentication.
4. Security settings: Check if your browser or device has any security settings that could be blocking access to the resource. Adjust them if needed.
5. System or network issues: If you are experiencing the error on multiple devices or networks, there may be a larger system issue. Contact your system administrator for assistance.
6. Training or account limitations: If you are using a specific software or service, make sure you have received proper training and that your account has the necessary privileges.
7. API or integration errors: If you are using third-party services or integrations, ensure that the necessary APIs and credentials are correctly configured.
Troubleshooting User Not Authorized Error
To troubleshoot the “User Not Authorized” error, follow these steps:
1. Check your account credentials: Make sure you are using the correct username and password to log in. If you are unsure, reset your password and try again.
2. Clear your browser history: On your mobile browser or desktop, open the settings and clear your browsing history and website data. This can often resolve authentication issues.
3. Check your device settings: Ensure that your device’s date and time are set correctly, as an incorrect time can cause authorization problems.
4. Log out and log back in: Sometimes, simply logging out of your account and logging back in can resolve authorization errors.
5. Use a different browser or device: If the issue persists, try accessing the service from a different web browser or device to see if the problem is specific to one platform.
If you continue to experience issues, reach out to our support team for further assistance.
Diagnosing and Resolving User Not Authorized Error
- Check user permissions: Ensure that the user has the necessary permissions to access the desired resource.
- Verify authentication credentials: Review the authentication credentials provided by the user and ensure they are correct.
- Validate access control settings: Check the access control settings for the user, resource, or application to ensure they are properly configured.
- Review user roles and groups: Confirm that the user is assigned to the appropriate roles and groups that grant access to the desired resource.
- Examine account status: Determine if the user account is active and not locked or disabled.
- Check network connectivity: Verify that the user has a stable and reliable network connection to access the resource.
- Review firewall and proxy settings: Ensure that any firewalls or proxy servers are not blocking the user’s access.
- Inspect application settings: Check the application settings to ensure they are correctly configured for user access.
- Investigate system or server issues: Look for any system or server problems that may be causing the user not authorized error.
- Consider user-specific issues: Take into account any specific circumstances or settings that may affect the user’s authorization status.
python
class UserNotAuthorizedError(Exception):
pass
def perform_authorized_action(user):
if not user.is_authorized():
raise UserNotAuthorizedError("User is not authorized to perform this action.")
# Code to perform the authorized action goes here
print("Action executed successfully.")
# Example usage
class User:
def __init__(self, username, is_authorized):
self.username = username
self.is_authorized = is_authorized
# Create an unauthorized user
unauthorized_user = User("Alice", False)
try:
perform_authorized_action(unauthorized_user)
except UserNotAuthorizedError as e:
print(f"Error: {str(e)}")
In the above code, we define a custom exception class `UserNotAuthorizedError`. The `perform_authorized_action` function checks if the user is authorized to execute a specific action. If the user is not authorized, a `UserNotAuthorizedError` is raised. The calling code can catch this exception and handle it accordingly.
Documented Solutions for User Not Authorized Error
Troubleshooting User Not Authorized Error
In this article, we will discuss some documented solutions for the “User Not Authorized” error that users may encounter.
Error Cause | Possible Solution |
---|---|
Incorrect credentials | 1. Double-check the username and password entered. 2. Reset the password if necessary. 3. Contact the system administrator to verify the account credentials. |
Insufficient privileges | 1. Check user permissions to ensure they have the necessary access rights. 2. Grant additional permissions if required. 3. Verify if the user is assigned to the correct user group or role. |
Expired or disabled account | 1. Check if the account has expired or been disabled. 2. Reactivate the account if necessary. 3. Verify if there are any account-related issues that might be causing the error. |
Network or server issues | 1. Verify network connectivity to ensure the user can reach the server. 2. Check server logs for any errors or issues. 3. Contact the system administrator to investigate any server-related problems. |
Software or application configuration | 1. Ensure the software or application is properly configured. 2. Check if any recent changes in the system have caused the error. 3. Reinstall or update the software if necessary. |
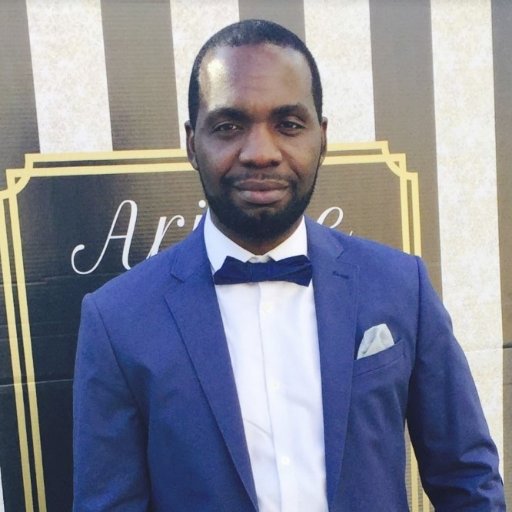